The Ultimate Guide to Solving Rust Compiler Issues: From `cargo` Updates to `rustc` Errors
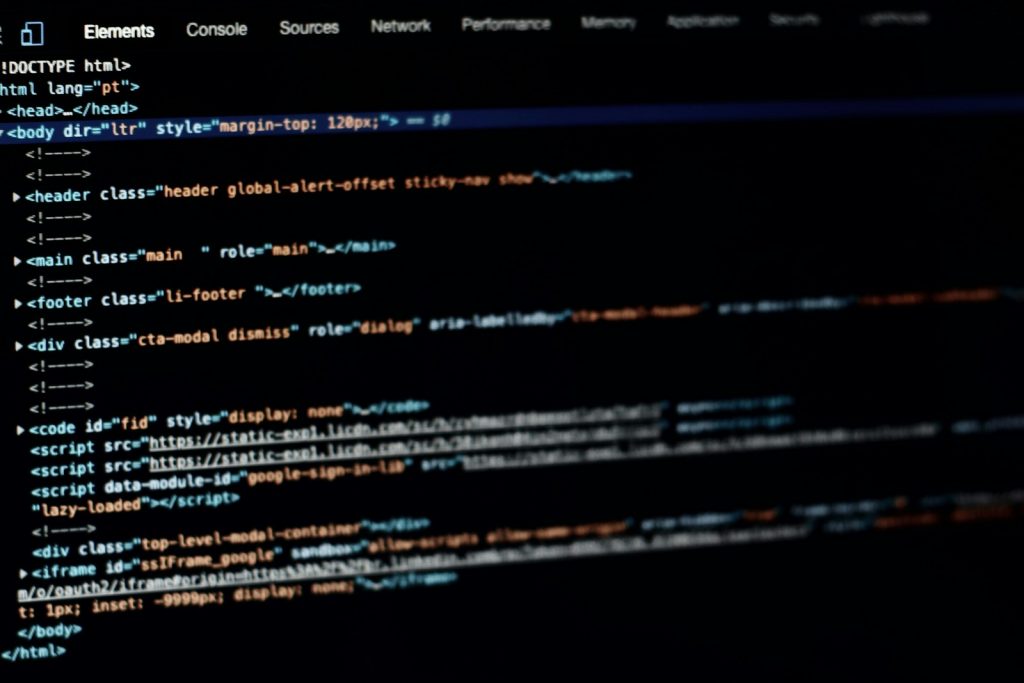
Introduction to Rust Compiler Challenges
Embarking on the journey of learning Rust can feel akin to scaling a steep mountain. Rust probably presents the most formidable learning curve among the plethora of programming languages, surpassing even that of C++. This ranking remains unchallenged by user experiences with languages such as x86-64 assembly, C, C#, Python, PHP, JavaScript, SQL, and others. The crux of Rust's complexity resides not in its syntax but in its stringent compiler restrictions, primarily geared towards enforced memory safety and concurrency without using a garbage collector. The strict compiler earns Rust its fame and notoriety; it ensures safety but demands precision, leading to errors for new developers around concepts like mutability, borrowing, and ownership.
Rust's difficulty arises from its unique approach to memory management. Unlike languages that offer more freedom in passing data around or rely on a runtime environment with a garbage collector to manage memory, Rust requires developers to engage deeply with how memory is allocated, used, and freed. This level of engagement is a double-edged sword; it eradicates a whole class of memory errors but at the cost of a steep initial learning curve and challenges in understanding and adhering to the compiler's demands.
Understanding Rust Compiler Basics
To truly grasp Rust and its compiler's workings, it's crucial to differentiate between some of its core concepts: moving, borrowing, and mutability. These mechanisms work collectively to uphold Rust's promises of memory safety and concurrency, but they also introduce a significant learning barrier.
- Moving refers to transferring ownership of a value from one variable to another. Unlike other languages where data is freely copied or referenced, Rust's move semantics mean that the original variable no longer has access to the value after the move.
- Borrowing, on the other hand, allows for the temporary use of a value through immutable or mutable references, enforcing at compile time that access is safe and does not lead to dangling pointers or data races.
- Mutability in Rust is explicit. Variables are immutable by default, promoting safety and predictability. Mutable access is granted explicitly and is tightly controlled by the borrowing rules to prevent unsafe modifications.
The Rust compiler enforces these rules with an iron fist. Attempting to skirt these restrictions will likely lead to the compiler flagging errors, preventing the code from compiling until these issues are resolved. While initially daunting, the errors serve as a powerful tool for learning Rust's safety features and understanding the reasons behind its design choices.
Understanding these basics sets the foundation for deeper dives into Rust's more complex features, such as lifetime specifiers and concurrency models, which rely on the same principles of ownership, borrowing, and mutability. Confronting the Rust is crucial in mastering the language and leveraging its capabilities to write safe, efficient, and concurrent code.
Common `rustc` Errors and How to Fix Them
When venturing into Rust, developers will inevitably encounter myriad errors emitted by `rustc`, Rust's compiler. These errors, far from being arbitrary, reflect the language's strict enforcement of rules around memory safety and concurrency. Understanding and resolving these errors is pivotal for successful compilation and deepening one's proficiency in Rust. Let's examine some of the common categories of errors and strategies to address them.
Dealing with Syntax and Compilation Errors
Syntax and compilation errors in Rust serve as the first line of defense against unsafe and incorrect code. Syntax errors are straightforward; they typically indicate typos or misunderstandings of the Rust syntax. Compilation errors, on the other hand, often point to deeper issues related to Rust's strict typing system, ownership, and borrowing mechanisms. It's crucial to read the error messages carefully to navigate these issues. Rust provides highly descriptive error messages designed to guide the programmer toward the source of the problem and suggest potential fixes.
Effective strategies for dealing with these errors include:
- Verifying the syntax against Rust's documentation or examples to catch any typos or misunderstandings.
- Utilizing tools like `rustfmt` to automatically format code according to Rust's style guidelines, helping to eliminate simple syntax errors.
- Breaking down complex expressions into smaller statements to isolate the cause of the error.
- Consulting the Rust community, including forums and chat channels, where many experienced developers can offer insights and solutions.
Understanding Ownership and Borrowing Issues
Ownership and borrowing issues represent a more advanced class of compile-time errors. These errors often stem from Rust's unique approach to memory management, where the compiler enforces strict rules to prevent memory leaks and data races. Common errors in this category include:
- Using a value after it has been moved.
- Attempting to mutate a value through an immutable reference.
- Violating lifetime constraints that ensure references are valid.
To resolve ownership and borrowing issues, developers can:
- Familiarize themselves with Rust's concepts of ownership, borrowing, and lifetimes, as a solid understanding of these is crucial for diagnosing and fixing related errors.
- Use explicit lifetimes for references when the compiler cannot infer them automatically. This can sometimes resolve confusing borrowing errors where the compiler's default assumptions do not match the developer's intentions.
- Refactor the code to reduce complexity, breaking down large functions into smaller ones that make ownership transfers and borrowing more explicit and manageable.
- Experiment with Rust's borrowing checker by intentionally creating small, controlled examples that produce errors and then resolving them. This practice can significantly improve one's understanding of how Rust manages memory.
By systematically addressing syntax, compilation, and ownership/borrowing issues, developers can fix their code and deepen their understanding of Rust's design principles. While sometimes frustrating, this learning process ultimately leads to mastery over one of the most powerful tools in the modern programmer's toolkit.
Navigating `cargo` Updates and Configuration
Working efficiently with Rust necessitates a keen understanding of `cargo`, its package manager and build system. `Cargo` is integral for managing Rust packages and configuring builds, making it a vital tool for Rust developers. Keeping `cargo` up-to-date and its dependencies allows developers to leverage the latest features and improvements, ensuring that projects remain efficient and secure.
How to Update Cargo and Manage Dependencies
Keeping `cargo` updated is straightforward and can be pivotal in maintaining the health of your Rust projects. To update `cargo`, simply run the command `rustup update` in your terminal. This command updates `rustc` (the Rust compiler) and `cargo`. Regularly running this command is recommended to keep your Rust toolchain current.
Managing dependencies with `cargo` involves carefully editing the `Cargo.toml` file, where project dependencies are specified. Adding a dependency is as simple as including the crate name and version in this file, and `cargo` takes care of the rest. Here are a few tips for managing dependencies efficiently:
- Use semantic versioning to ensure you receive updates that maintain backward compatibility. This is done by specifying dependencies using the caret syntax, e.g., crate_name = "^1.2.3".
- Periodically review and update the dependencies listed in `Cargo.toml` to their latest versions to take advantage of performance improvements, new features, and security patches.
- Consider using `cargo-outdated` to check for outdated dependencies within your project. Install this tool using cargo install cargo-outdated and run cargo outdated to see a list of dependencies with newer versions available.
By adhering to these practices, developers can ensure that their Rust projects are built on a stable, up-to-date foundation that maximizes performance and security.
Resolving Common Cargo Warnings and Errors
In developing Rust projects, encountering warnings and errors from `cargo` is inevitable. Many of these warnings are intended to guide developers away from potential issues or deprecated practices. Here are strategies to resolve some of the most common `cargo` warnings and errors:
- Warnings about deprecated syntax or features: The Rust ecosystem evolves rapidly, and it's common for previously valid syntax or features to become deprecated. Resolve these warnings by referring to the Rust documentation or the warning details to update your code with the recommended syntax or features.
- Errors due to missing dependencies or mismatched versions: Ensure that all dependencies are correctly listed in `Cargo.toml` and that their versions are compatible. If you're facing version conflicts, consider using the `cargo tree` command to inspect dependency trees and identify sources of conflict.
- Failures related to `Cargo.toml` or `Cargo.lock`: These files are crucial for managing project configurations and ensuring reproducible builds. If `cargo build` fails due to issues in these files, check for syntax errors in `Cargo.toml` and consider running `cargo update` to refresh `Cargo.lock` with the latest compatible dependency versions.
Advanced usage of Cargo might involve configuring build scripts or opting into features that can lead to more complex warnings and errors. In such cases, deeper documentation perusal and community engagement can provide resolutions. As a last resort for unresolved dependency conflicts, manually editing the `Cargo.lock` file might be necessary but is generally discouraged without thoroughly understanding the dependencies involved.
Navigating `cargo` updates and configurations is essential for Rust developers aiming to maximize efficiency and maintain the integrity of their projects. Developers can harness Cargo's full potential to streamline its Rust development workflow through regular maintenance and adherence to best practices.
Advanced Techniques for Debugging Rust Code
Debugging Rust code, while initially daunting due to the compiler's strictness, can be markedly streamlined with a combination of advanced tooling and leveraging the knowledge base of the Rust community. Employing the right tools and resources can transform a frustrating debugging session into a series of logical steps toward code correction and optimization.
Using Rust Analyzer and Other Tooling
Rust Analyzer is one of the most powerful tools at a Rust developer's disposal for code analysis and debugging. Integrated into editors like VSCode, Vim, and Emacs, Rust Analyzer provides real-time code analysis, offering quick fixes, autocompletion, and in-depth documentation directly in the editor. This immediate feedback loop can prevent common errors before compilation and suggest potential solutions to detected issues.
Beyond Rust Analyzer, the Rust ecosystem offers other robust tooling options for debugging, such as:
- lldb and gdb: Command-line debuggers that can inspect the state of a program while it's running, allowing for breakpoints, step-through execution, and variables inspection.
- cargo-watch: A tool that watches over your project's files for changes and automatically compiles your project or runs tests, ensuring immediate feedback on the impact of code modifications.
- cargo-expand: Useful for macro expansion, it helps understand how macros are transformed into Rust code, clarifying compiler errors that originate from macro use.
Effectively utilizing these tools enables developers to delve deeper into their code's behavior and uncover the root causes of persistent bugs.
Leveraging Community Resources for Troubleshooting
The Rust community is known for its willingness to help and a plethora of resources tailored to problem-solving. When dealing with particularly stubborn bugs or complex code issues, these resources can be invaluable:
- The Rust Users Forum and Rust Subreddit: Platforms where Rust programmers of all skill levels share advice, solutions, and general support.
- Stack Overflow: Contains a vast library of Rust-related questions and answers, which can offer insight into problems similar to the ones you're facing.
- Rust Documentation: The official Rust documentation and books like "The Rust Programming Language" and "Rust by Example" provide comprehensive guides on Rust's features and best practices.
- Rust Internals Forum: For understanding the deeper aspects of Rust and engaging with ongoing discussions about the language's development.
Engaging with these resources not only aids in immediate problem-solving but also contributes to a deeper understanding of Rust, its compiler, and best practices for efficient coding. Whether debugging alone with the help of advanced tooling or seeking out the collective wisdom of the Rust community, developers are never without options for troubleshooting and learning.
Mastering the debugging process in Rust involves leveraging its powerful tool ecosystem and actively engaging with the community. Through these combined approaches, developers can overcome the challenges posed by Rust's stringent compiler and ultimately craft more reliable, efficient, and safe software.
Best Practices for Avoiding Rust Compiler Issues
Encountering Rust compiler issues can seem inevitable, particularly for those new to the language. Yet, with a strategic approach centered on understanding Rust's core principles and leveraging its type system, many common issues can be avoided. Following best practices in code writing, structuring, and testing minimizes compiler errors and enhances code maintainability and efficiency.
Writing Clean and Maintainable Rust Code
Writing clean and maintainable code in Rust involves deeply engaging with the language's unique features, such as ownership, borrowing, and lifetimes. These features, while at first may seem like obstacles, are, in fact, powerful tools designed to enforce memory safety and concurrency correctness at compile time. By embracing these concepts and adhering to idiomatic Rust practices, developers can write robust applications that fully leverage Rust's performance and safety guarantees.
- Favor explicit over implicit: Rust's type system and ownership model encourage being explicit about data types and how values are passed around in your program. This makes your code clearer to readers and helps the compiler catch potential issues early.
- Utilize type aliases: Creating type aliases can significantly enhance code readability and maintainability for complex or commonly used types.
- Make use of enums and pattern matching: Rust's enums and exhaustive pattern matching are powerful features that can express complex state distinctions and associated data clearly and concisely, improving both safety and expressiveness.
- Leverage the compiler's checks: The Rust compiler is invaluable for enforcing code correctness. Paying close attention to warning and error messages and understanding the underlying principles being enforced can guide you in writing better code.
- Refactor with ownership in mind: Rust's ownership model is unique and often requires a shift in thinking from other languages. Structuring your code to clearly define ownership and borrowing can help avoid common pitfalls such as dangling pointers or data races.
- Adopt a testing mindset: Writing unit tests and leveraging Rust's integrated testing framework can catch errors early and ensure your code behaves as expected as it evolves.
- Use `rustfmt` and `clippy`: Regularly running these tools on your codebase ensures consistency with Rust's style guidelines and can catch common mistakes and inefficiencies.
Adopting these practices requires patience and consistent effort, especially for those coming from different programming paradigms. However, the payoff regarding code quality, safety, and performance can be significant. As you become more familiar with Rust's idioms and patterns, the compiler becomes more of an ally than an adversary, guiding you toward writing clean, efficient, and maintainable Rust code.
Conclusion: Mastering Rust Compiler Troubleshooting
The journey from encountering your first Rust compiler error to fluently navigating and resolving such issues is a testament to both the complexity and the power of the Rust programming language. Mastering Rust compiler troubleshooting is not merely about overcoming the learning curve; it's about embracing a mindset prioritizing safety, efficiency, and clarity in software development. The path to proficiency is paved with challenges, from deciphering the nuances of ownership and borrowing to leveraging advanced tooling for debugging. However, each obstacle overcome enriches your understanding and harnesses the full potential of Rust.
Success in Rust is measured by the ability to write code that satisfies the compiler and by recognizing the compiler as a vital tool that guides toward best practices and robust software design. Learning to work harmoniously with the Rust compiler can be demanding. Still, it is ultimately rewarding, offering guarantees about the safety and reliability of your code that are hard to find in other programming environments.
As developers deepen their engagement with Rust, adopting best practices for code writing and structuring, they uncover the intrinsic benefits of the language. This includes confidence in building concurrent and memory-safe applications and the efficiency gained from leveraging Rust's powerful type system and zero-cost abstractions. Moreover, the vibrant Rust community stands as a pillar of support, offering guidance, resources, and encouragement to newcomers and seasoned programmers alike.
In conclusion, mastering the intricacies of Rust compiler troubleshooting is an ongoing process that extends beyond technical skills. It encompasses a philosophical alignment with the language's safety and performance goals. With time, practice, and a proactive approach to learning, navigating Rust's compiler becomes manageable and a source of professional growth and satisfaction. As the Rust language and its ecosystem evolve, so will the strategies for effective troubleshooting, ensuring that Rust remains at the forefront of safe and efficient system-level programming.